The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. It is often used to provide a default value for a variable or to check if a variable is null or undefined.
The double question mark operator is a powerful tool that can be used to improve the readability and maintainability of your code. It can also help to prevent errors by ensuring that variables are always assigned a valid value.
Here are some of the benefits of using the double question mark operator:
- Improved readability and maintainability
- Reduced risk of errors
- More concise code
If you are not already using the double question mark operator, I encourage you to start using it today. It is a simple and powerful tool that can make your code more readable, maintainable, and error-free.
JavaScript Double Question Mark Operator (??)
The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. It is often used to provide a default value for a variable or to check if a variable is null or undefined.
- Logical Operator: ?? is a logical operator that evaluates two operands.
- Truthy/Falsy: It returns the first operand if it is truthy, and the second operand if it is falsy.
- Default Value: ?? can be used to provide a default value for a variable.
- Null/Undefined Check: ?? can be used to check if a variable is null or undefined.
- Concise Code: ?? can help to write more concise code.
- Readable Code: ?? can improve the readability of code.
- Maintainable Code: ?? can help to write more maintainable code.
- Error Prevention: ?? can help to prevent errors by ensuring that variables are always assigned a valid value.
The double question mark operator is a powerful tool that can be used to improve the quality of your JavaScript code. It is a simple and easy-to-use operator that can save you time and effort in the long run.
Logical Operator
The JavaScript double question mark operator (??) is a logical operator, meaning that it evaluates two operands and returns a single value. The operands are evaluated from left to right, and the operator returns the value of the first operand if it is truthy, or the value of the second operand if the first operand is falsy.
This operator is often used to provide a default value for a variable. For example, the following code snippet assigns the value of the variable `x` to the variable `y`, or the value `0` if `x` is null or undefined:
jslet y = x ?? 0;
The double question mark operator can also be used to check if a variable is null or undefined. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:
if (x ?? null) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code.
Conclusion
The double question mark operator is a versatile operator that can be used for a variety of purposes. It is a valuable tool for any JavaScript developer, and it can help to improve the quality of your code.
Truthy/Falsy
The truthy/falsy nature of the double question mark operator is one of its most important features. It allows the operator to be used for a variety of purposes, including providing default values and checking for null or undefined values.
When the first operand of the double question mark operator is truthy, the operator returns the first operand. This means that the first operand can be used to provide a default value for the second operand. For example, the following code snippet assigns the value of the variable `x` to the variable `y`, or the value `0` if `x` is null or undefined:
jslet y = x ?? 0;
In this example, the first operand (`x`) is truthy if it is not null or undefined. If `x` is not null or undefined, then the value of `x` is assigned to `y`. Otherwise, the value `0` is assigned to `y`.
When the first operand of the double question mark operator is falsy, the operator returns the second operand. This means that the second operand can be used to provide a fallback value for the first operand. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:
if (x ?? null) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
In this example, the first operand (`x`) is falsy if it is null or undefined. If `x` is null or undefined, then the message `”x is null or undefined”` is logged to the console. Otherwise, the message `”x is not null or undefined”` is logged to the console.
The truthy/falsy nature of the double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code.
Conclusion
The truthy/falsy nature of the double question mark operator is one of its most important features. It allows the operator to be used for a variety of purposes, including providing default values and checking for null or undefined values. Understanding how the truthy/falsy nature of the double question mark operator works is essential for using the operator effectively.
Default Value
The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. This makes it a powerful tool for providing default values for variables.
-
Default Values for Function Parameters
The double question mark operator can be used to provide default values for function parameters. This can be useful for making your functions more flexible and easier to use. For example, the following function takes a parameter `x` with a default value of `0`:jsfunction add(x = 0, y) { return x + y;}
This function can be called with one or two arguments. If only one argument is provided, the default value of `0` will be used for the `x` parameter.
-
Default Values for Object Properties
The double question mark operator can also be used to provide default values for object properties. This can be useful for ensuring that objects always have a valid value for a particular property. For example, the following object has a `name` property with a default value of `”John Doe”`:jsconst person = { name: “John Doe” ?? null,};
If the `name` property is not set to a specific value, the default value of `”John Doe”` will be used.
-
Default Values for Array Elements
The double question mark operator can also be used to provide default values for array elements. This can be useful for ensuring that arrays always have a valid value at a particular index. For example, the following array has a default value of `0` for all elements:jsconst numbers = new Array(10).fill(0 ?? null);
If an element of the `numbers` array is not set to a specific value, the default value of `0` will be used.
-
Default Values for Variables
The double question mark operator can also be used to provide default values for variables. This can be useful for ensuring that variables always have a valid value. For example, the following variable has a default value of `0`:jslet x = 0 ?? null;
If the `x` variable is not set to a specific value, the default value of `0` will be used.
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code. By providing default values for variables, you can help to ensure that your code always behaves as expected.
Null/Undefined Check
The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. This makes it a powerful tool for checking if a variable is null or undefined.
-
Preventing Errors
One of the most common uses of the double question mark operator is to prevent errors. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:jsif (x ?? null) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
This code snippet can be used to prevent errors that may occur if the `x` variable is null or undefined. For example, the following code snippet will throw an error if the `x` variable is null or undefined:
jsconsole.log(x.toString());
However, the following code snippet will not throw an error if the `x` variable is null or undefined:
jsconsole.log((x ?? null).toString());
-
Improving Readability
The double question mark operator can also be used to improve the readability of your code. For example, the following code snippet is difficult to read and understand:jsif (x !== null && x !== undefined) { // do something}
The following code snippet is much easier to read and understand:
jsif (x ?? null) { // do something}
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code. By using the double question mark operator to check if a variable is null or undefined, you can help to prevent errors and improve the quality of your code.
Concise Code
The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. This makes it a powerful tool for writing more concise code.
One of the most common uses of the double question mark operator is to provide default values for variables. For example, the following code snippet assigns the value of the variable `x` to the variable `y`, or the value `0` if `x` is null or undefined:
jslet y = x ?? 0;
This code snippet is much more concise than the following code snippet:
jsif (x !== null && x !== undefined) { y = x;} else { y = 0;}
The double question mark operator can also be used to check if a variable is null or undefined. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:
jsif (x ?? null) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
This code snippet is much more concise than the following code snippet:
jsif (x !== null && x !== undefined) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code. By using the double question mark operator to write more concise code, you can save time and effort in the long run.
Conclusion
The double question mark operator is a valuable tool for writing more concise JavaScript code. By understanding how to use the double question mark operator effectively, you can improve the quality of your code and save time and effort in the long run.
Readable Code
The JavaScript double question mark operator (??) is a logical operator that returns the first operand if it is truthy, and the second operand if it is falsy. This makes it a powerful tool for improving the readability of code.
One of the most common uses of the double question mark operator is to provide default values for variables. For example, the following code snippet assigns the value of the variable `x` to the variable `y`, or the value `0` if `x` is null or undefined:
jslet y = x ?? 0;
This code snippet is much more readable than the following code snippet:
jsif (x !== null && x !== undefined) { y = x;} else { y = 0;}
The double question mark operator can also be used to check if a variable is null or undefined. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:
jsif (x ?? null) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
This code snippet is much more readable than the following code snippet:
jsif (x !== null && x !== undefined) { console.log(“x is not null or undefined”);} else { console.log(“x is null or undefined”);}
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and error handling of your JavaScript code. By using the double question mark operator to write more readable code, you can make your code easier to read and understand, which can save you time and effort in the long run.
Conclusion
The double question mark operator is a valuable tool for writing more readable JavaScript code. By understanding how to use the double question mark operator effectively, you can improve the quality of your code and make it easier for others to read and understand.
Maintainable Code
In the realm of software development, maintainability stands as a cornerstone of quality code. It’s the ability of code to be easily understood, modified, and extended over its lifetime. The JavaScript double question mark operator (??) plays a significant role in enhancing the maintainability of code.
-
Simplified Code Structure
The double question mark operator simplifies code structure by providing a concise syntax for handling null and undefined values. It eliminates the need for lengthy conditional statements or explicit checks, making the code more readable and easier to follow. -
Reduced Code Duplication
By providing a default value for variables, the double question mark operator helps reduce code duplication. Instead of repeating the same assignment or checking code in multiple places, developers can rely on the operator to ensure a consistent behavior. -
Improved Error Handling
The double question mark operator aids in error handling by providing a graceful way to deal with null and undefined values. It allows developers to handle these situations explicitly, preventing unexpected errors and ensuring the robustness of the code. -
Enhanced Code Readability
The double question mark operator improves code readability by making the code more concise and self-explanatory. Developers can quickly identify the intended behavior and the handling of null and undefined values, without the need for additional comments or documentation.
In summary, the JavaScript double question mark operator (??) is a powerful tool that contributes to the maintainability of code. By simplifying code structure, reducing code duplication, improving error handling, and enhancing code readability, the operator empowers developers to write code that is easier to understand, modify, and extend in the long run.
Error Prevention
In the realm of JavaScript development, the double question mark operator (??) stands as a formidable ally in the battle against errors. It empowers developers to assign a fallback value to variables, mitigating the perils of null and undefined values that can wreak havoc upon the smooth execution of code.
-
Enforced Initialization
The double question mark operator enforces the initialization of variables, ensuring they are never left in a state of limbo where their values are undefined. By providing a default value, the operator prevents the code from encountering unexpected errors that may arise from attempting to access or manipulate uninitialized variables. -
Consistent Data Handling
The double question mark operator promotes consistent data handling by ensuring that variables always contain a valid value. This consistency safeguards against scenarios where the absence of a value could lead to erroneous results or unpredictable behavior. It establishes a solid foundation for reliable code execution. -
Improved Code Readability
The double question mark operator enhances code readability by making it immediately apparent that a variable has a fallback value. This clarity reduces the cognitive load for developers, allowing them to quickly grasp the intended behavior of the code without getting bogged down in complex conditional statements. -
Reduced Debugging Time
By proactively preventing errors through value assignment, the double question mark operator significantly reduces debugging time. Developers can spend less effort tracking down elusive bugs caused by uninitialized variables and more time focusing on the core functionality of their applications.
In conclusion, the double question mark operator (??) serves as an invaluable tool for error prevention in JavaScript development. It ensures that variables are always assigned a valid value, fostering robust and reliable code. By embracing the use of this operator, developers can minimize the occurrence of errors, enhance code readability, and accelerate the debugging process, ultimately leading to a higher quality of software.
FAQs about the JavaScript Double Question Mark Operator (??)
The JavaScript double question mark operator (??) is a powerful tool that can be used to improve the readability, maintainability, and error handling of your code. It can be used to provide default values for variables, check if a variable is null or undefined, and write more concise and readable code. Here are some frequently asked questions about the double question mark operator:
Question 1: What is the difference between the double question mark operator (??) and the logical OR operator (||)?
The double question mark operator (??) returns the first operand if it is truthy, and the second operand if it is falsy. The logical OR operator (||) returns the first operand if it is truthy, and the second operand only if the first operand is falsy. This means that the double question mark operator can be used to provide default values for variables, while the logical OR operator cannot.
Question 2: When should I use the double question mark operator (??)?
The double question mark operator (??) can be used in a variety of situations, including:
- Providing default values for variables
- Checking if a variable is null or undefined
- Writing more concise and readable code
Question 3: What are the benefits of using the double question mark operator (??)?
The double question mark operator (??) has a number of benefits, including:
- Improved readability and maintainability
- Reduced risk of errors
- More concise code
Question 4: Are there any drawbacks to using the double question mark operator (??)?
The double question mark operator (??) can be slightly more difficult to read and understand than the logical OR operator (||). However, this drawback is outweighed by the benefits of using the double question mark operator.
Question 5: What are some examples of how to use the double question mark operator (??)?
Here are some examples of how to use the double question mark operator (??):
-
const name = user?.name ?? "John Doe";
-
if (user?.age) { ... }
-
const numbers = new Array(10).fill(0 ?? null);
Summary
The double question mark operator (??) is a powerful tool that can be used to improve the quality of your JavaScript code. It is a simple and easy-to-use operator that can save you time and effort in the long run.
Transition to the next article section
Now that you have a basic understanding of the double question mark operator (??), you can learn more about its advanced features and use cases in the next section.
Tips for Using the JavaScript Double Question Mark Operator (??)
The JavaScript double question mark operator (??) is a powerful tool that can be used to improve the quality of your code. Here are some tips for using the double question mark operator effectively:
Tip 1: Use the double question mark operator to provide default values for variables.
This can help to prevent errors and make your code more readable. For example, the following code snippet assigns the value of the variable `x` to the variable `y`, or the value `0` if `x` is null or undefined:
jslet y = x ?? 0;
Tip 2: Use the double question mark operator to check if a variable is null or undefined.
This can be useful for preventing errors and improving the readability of your code. For example, the following code snippet logs the message `”x is null or undefined”` to the console if the variable `x` is null or undefined:
jsif (x ?? null) {console.log(“x is not null or undefined”);} else {console.log(“x is null or undefined”);}
Tip 3: Use the double question mark operator to write more concise code.
The double question mark operator can be used to write more concise code by providing a default value for a variable in a single line of code. For example, the following code snippet is equivalent to the previous example:
jslet y = (x ?? 0);
Tip 4: Use the double question mark operator to improve the readability of your code.
The double question mark operator can be used to make your code more readable by making it clear that a variable has a default value. For example, the following code snippet is more readable than the previous example:
jslet y = x ?? 0; // y is assigned the value of x if x is not null or undefined, otherwise y is assigned the value 0
Tip 5: Use the double question mark operator to reduce the risk of errors.
The double question mark operator can be used to reduce the risk of errors by ensuring that variables are always assigned a valid value. For example, the following code snippet will not throw an error if the variable `x` is null or undefined:
jsconsole.log((x ?? 0).toString());
Summary
The double question mark operator is a powerful tool that can be used to improve the quality of your JavaScript code. By following these tips, you can use the double question mark operator effectively to write more readable, maintainable, and error-free code.
Conclusion
The JavaScript double question mark operator (??) is a powerful tool that can be used to improve the readability, maintainability, and error handling of your code. It is a simple and easy-to-use operator that can save you time and effort in the long run.
This article has explored the various uses of the double question mark operator, including providing default values for variables, checking if a variable is null or undefined, and writing more concise and readable code. We have also provided some tips for using the double question mark operator effectively.
As you continue to develop your JavaScript skills, we encourage you to experiment with the double question mark operator and see how it can improve the quality of your code. By understanding how to use the double question mark operator effectively, you can write more readable, maintainable, and error-free code.
Youtube Video:
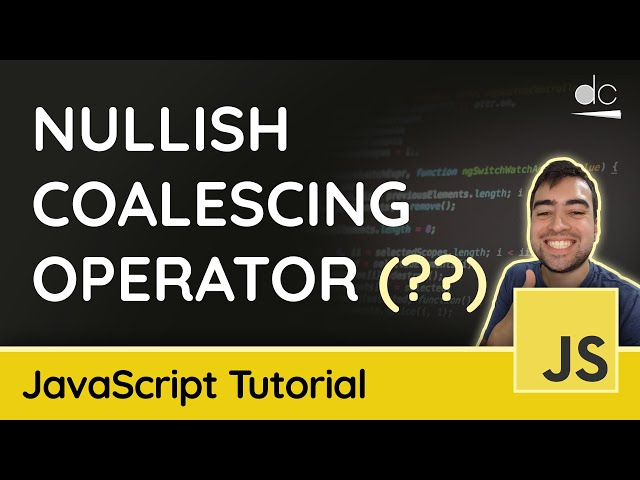