In JavaScript, the double question mark (??) is a logical operator that evaluates its left operand and returns its right operand only if the left operand is null or undefined. This operator is often used as a replacement for the ternary conditional operator (?:) when the goal is to provide a default value for a variable or property.
The double question mark operator is particularly useful in situations where the left operand may be undefined or null due to asynchronous operations or external factors. By using the ?? operator, you can ensure that a default value is always returned, preventing errors and ensuring the smooth execution of your code.
Here are some of the key benefits of using the double question mark operator:
- Improved code readability and maintainability
- Simplified error handling
- Enhanced null and undefined checking
Overall, the double question mark operator is a valuable tool in the JavaScript developer’s toolkit. It provides a concise and efficient way to handle undefined and null values, ensuring the smooth execution of your code and improving its overall quality.
double question mark js
The double question mark (??) in JavaScript is a logical operator that evaluates its left operand and returns its right operand only if the left operand is null or undefined. It is often used as a replacement for the ternary conditional operator (?:) when the goal is to provide a default value for a variable or property.
-
Syntax:
??
- Associativity: Right-to-left
- Precedence: 10 (same as the ternary conditional operator)
- Purpose: Nullish coalescing
- Benefits: Improved code readability and maintainability, simplified error handling, enhanced null and undefined checking
-
Example:
const name = user?.name ?? "Guest";
- Comparison to ternary conditional operator: The double question mark operator is more concise and easier to read than the ternary conditional operator, especially when the default value is simple.
- Use cases: The double question mark operator is particularly useful in situations where the left operand may be undefined or null due to asynchronous operations or external factors.
- Alternatives: In some cases, the double question mark operator can be replaced with the logical OR (||) operator, but the double question mark operator is more concise and expressive.
Overall, the double question mark operator is a valuable tool in the JavaScript developer’s toolkit. It provides a concise and efficient way to handle undefined and null values, ensuring the smooth execution of your code and improving its overall quality.
Syntax
The syntax of the double question mark operator in JavaScript is ??
. This operator takes two operands: a left operand and a right operand. The left operand is evaluated first, and if it is null or undefined, the right operand is returned. Otherwise, the left operand is returned. For example, the following code returns “Guest” if the user object does not have a name property:
const user = { name: undefined };const name = user?.name ?? "Guest";console.log(name); // Guest
The double question mark operator is often used as a replacement for the ternary conditional operator (?:) when the goal is to provide a default value for a variable or property. However, the double question mark operator is more concise and easier to read, especially when the default value is simple. For example, the following two code snippets are equivalent:
// Using the ternary conditional operatorconst name = user ? user.name : "Guest";// Using the double question mark operatorconst name = user?.name ?? "Guest";
The double question mark operator is a valuable tool in the JavaScript developer’s toolkit. It provides a concise and efficient way to handle undefined and null values, ensuring the smooth execution of your code and improving its overall quality.
Associativity
The double question mark operator in JavaScript is right-associative, meaning that it evaluates its operands from right to left. This is in contrast to the left-associative ternary conditional operator (?:), which evaluates its operands from left to right. The right-associativity of the double question mark operator can be seen in the following example:
const user = { name: "John Doe" };const name = user?.name ?? user?.email ?? "Guest";console.log(name); // John Doe
In this example, the double question mark operator first evaluates the expression user?.name
. Since user.name
is not null or undefined, it returns “John Doe”. The double question mark operator then evaluates the expression user?.email
, but since user.email
is null or undefined, it returns “Guest”. Finally, the double question mark operator returns “John Doe” because it is the first non-null or undefined value.
The right-associativity of the double question mark operator can be useful in situations where you want to chain multiple default values. For example, the following code snippet returns the first non-null or undefined value from the array names
:
const names = ["John Doe", null, "Jane Doe"];const name = names[0] ?? names[1] ?? names[2] ?? "Guest";console.log(name); // John Doe
The right-associativity of the double question mark operator ensures that the default value is only returned if all of the preceding values are null or undefined.
Overall, the right-associativity of the double question mark operator is an important aspect of its behavior. It allows you to chain multiple default values and ensures that the default value is only returned if all of the preceding values are null or undefined.
Precedence
The precedence of the double question mark operator in JavaScript is 10, which is the same as the precedence of the ternary conditional operator (?:). This means that the double question mark operator and the ternary conditional operator have the same binding power, and they are evaluated from right to left. This can be seen in the following example:
const user = { name: "John Doe" };const name = user?.name ?? user?.email ?? "Guest";console.log(name); // John Doe
In this example, the double question mark operator is used to chain multiple default values. The first default value is “John Doe”, and the second default value is “Guest”. Since the user object has a name property, the first default value is returned. However, if the user object did not have a name property, the second default value would have been returned.
The precedence of the double question mark operator is important because it determines the order in which it is evaluated relative to other operators. In the example above, the double question mark operator is evaluated after the ?. operator. This is because the ?. operator has a higher precedence than the double question mark operator. As a result, the ?. operator is evaluated first, and the double question mark operator is evaluated second.
Understanding the precedence of the double question mark operator is important for writing JavaScript code that is correct and efficient. By understanding the precedence of the double question mark operator, you can avoid errors and ensure that your code executes as expected.
Purpose
The double question mark operator (??) in JavaScript is specifically designed for nullish coalescing, which refers to the process of returning a default value if the left operand is null or undefined. This behavior is distinct from the logical OR (||) operator, which returns the first truthy value or the last value if all operands are falsy. Nullish coalescing is particularly useful in situations where you want to handle null and undefined values explicitly, ensuring that a default value is always returned.
For example, consider the following code snippet:
const user = { name: undefined };const name = user?.name ?? "Guest";
In this example, the double question mark operator is used to assign a default value (“Guest”) to the name variable if the user object’s name property is null or undefined. This prevents errors and ensures that the name variable always contains a valid value.
Nullish coalescing is a valuable tool in JavaScript development, as it provides a concise and efficient way to handle null and undefined values. By understanding the purpose of nullish coalescing and how it is implemented in the double question mark operator, you can write more robust and maintainable code.
Benefits
The double question mark operator (??) in JavaScript offers several key benefits that enhance the quality and maintainability of your code:
-
Improved code readability and maintainability
The double question mark operator provides a concise and expressive way to handle null and undefined values. It eliminates the need for lengthy if-else statements or ternary conditional operators, making your code more readable and easier to maintain. -
Simplified error handling
The double question mark operator simplifies error handling by providing a default value in case the left operand is null or undefined. This prevents errors from being thrown and ensures the smooth execution of your code. -
Enhanced null and undefined checking
The double question mark operator provides a more robust and consistent way to check for null and undefined values compared to traditional methods. It ensures that null and undefined values are handled explicitly, reducing the risk of errors and unexpected behavior.
Overall, the double question mark operator is a valuable tool that enhances the readability, maintainability, and robustness of your JavaScript code. By utilizing this operator effectively, you can write code that is more reliable, easier to understand, and less prone to errors.
Example
The example const name = user?.name ?? "Guest";
showcases the practical application of the double question mark operator (??) in JavaScript. It demonstrates how to assign a default value (“Guest”) to the name
variable if the user.name
property is null
or undefined
. This technique is commonly used to handle missing or undefined properties in objects, ensuring that a valid value is always available.
The double question mark operator is particularly useful in situations where you need to access nested properties or perform complex operations on objects. By using the double question mark operator, you can avoid potential errors and ensure that your code executes smoothly, even when dealing with missing or undefined values.
In summary, the example const name = user?.name ?? "Guest";
provides a practical demonstration of how the double question mark operator can be used to handle null and undefined values in JavaScript. This technique is essential for writing robust and maintainable code, especially when working with complex data structures and asynchronous operations.
Comparison to ternary conditional operator
The double question mark operator (??) in JavaScript is often compared to the ternary conditional operator (?:) because of their similar functionality in providing a default value if a certain condition is met. However, the double question mark operator has several advantages over the ternary conditional operator, particularly in terms of conciseness and readability.
-
Conciseness: The double question mark operator is more concise than the ternary conditional operator, especially when the default value is simple. For example, the following code snippet uses the double question mark operator to assign a default value of “Guest” to the name variable if the user object’s name property is null or undefined:
const name = user?.name ?? "Guest";
This is equivalent to the following code snippet using the ternary conditional operator:
const name = user.name ? user.name : "Guest";
As you can see, the double question mark operator is more concise and easier to read, especially when the default value is simple.
- Readability: The double question mark operator is also easier to read than the ternary conditional operator, especially for developers who are new to JavaScript. The double question mark operator’s syntax is more straightforward and intuitive, making it easier to understand what the code is doing.
Overall, the double question mark operator is a more concise and easier to read alternative to the ternary conditional operator, especially when the default value is simple. This makes it a valuable tool for writing JavaScript code that is both efficient and maintainable.
Use cases
The double question mark operator (??) in JavaScript is particularly useful in situations where the left operand may be undefined or null due to asynchronous operations or external factors. This is because the double question mark operator returns the right operand only if the left operand is null or undefined, providing a default value in these situations.
Asynchronous operations are operations that do not complete immediately and may take some time to finish. During this time, the left operand may be undefined or null, and the double question mark operator ensures that a default value is returned to prevent errors and ensure the smooth execution of the code.
External factors can also cause the left operand to be undefined or null. For example, if data is being fetched from a server, the response may be delayed or may not contain the expected data. In these cases, the double question mark operator can be used to provide a default value and handle the missing or incomplete data gracefully.
A practical example of using the double question mark operator to handle asynchronous operations is when fetching data from an API. The following code snippet shows how to use the double question mark operator to assign a default value to the data
variable if the API response is null or undefined:
const data = await fetch('https://example.com/api/data').then(response => response.json()) ?? [];
In this example, the fetch
function returns a Promise, which is an asynchronous operation. The then
method is used to convert the Promise to a resolved value, which is the JSON response from the API. The double question mark operator is then used to assign a default value of an empty array to the data
variable if the API response is null or undefined.
By understanding the connection between the double question mark operator and its use cases in handling asynchronous operations and external factors, developers can write more robust and maintainable JavaScript code that can handle missing or incomplete data gracefully.
Alternatives
The double question mark operator (??) in JavaScript is a concise and expressive alternative to the logical OR (||) operator when the goal is to provide a default value for a variable or property. However, there are some key differences between the two operators that make the double question mark operator more suitable in certain situations.
The logical OR (||) operator returns the first truthy value in a series of operands. If all operands are falsy, it returns the last operand. This means that the logical OR operator can be used to provide a default value, but it will return the default value even if the left operand is null or undefined.
The double question mark operator, on the other hand, only returns the right operand if the left operand is null or undefined. This makes it more suitable for situations where you want to explicitly handle null and undefined values.
For example, consider the following code snippet:
const name = user || "Guest";
In this example, the logical OR operator will return “Guest” if the user object does not have a name property, even if the name property is explicitly set to null or undefined.
const name = user?.name ?? "Guest";
In this example, the double question mark operator will only return “Guest” if the user object does not have a name property and the name property is explicitly set to null or undefined.
As you can see, the double question mark operator provides a more explicit and controlled way to handle null and undefined values. This makes it a valuable tool for writing robust and maintainable JavaScript code.
FAQs on the Double Question Mark Operator (??) in JavaScript
This section addresses frequently asked questions about the double question mark operator (??) in JavaScript, providing clear and informative answers to common concerns and misconceptions.
Question 1: What is the purpose of the double question mark operator (??)?
Answer: The double question mark operator is used for nullish coalescing. It returns the right operand if the left operand is null or undefined, providing a default value in these situations.
Question 2: How does the double question mark operator differ from the logical OR (||) operator?
Answer: The logical OR operator returns the first truthy value, while the double question mark operator only returns the right operand if the left operand is null or undefined. This makes the double question mark operator more suitable for explicitly handling null and undefined values.
Question 3: When should I use the double question mark operator?
Answer: The double question mark operator should be used when you want to provide a default value for a variable or property, especially when you need to explicitly handle null and undefined values.
Question 4: Are there any alternatives to the double question mark operator?
Answer: In some cases, the logical OR (||) operator can be used as an alternative, but the double question mark operator is more concise and expressive, especially when handling null and undefined values.
Question 5: What are the benefits of using the double question mark operator?
Answer: The double question mark operator improves code readability, simplifies error handling, and enhances null and undefined checking, making your code more robust and maintainable.
Question 6: Is the double question mark operator supported in all JavaScript environments?
Answer: Yes, the double question mark operator is supported in all modern JavaScript environments, including browsers and Node.js.
Summary: The double question mark operator is a powerful tool for handling null and undefined values in JavaScript. It provides a concise and expressive way to assign default values and enhance the robustness of your code. By understanding the purpose, usage, and benefits of the double question mark operator, you can write more effective and maintainable JavaScript applications.
Transition to Next Article Section: This concludes our discussion on the double question mark operator. In the next section, we will explore advanced techniques for working with data in JavaScript.
Tips for Using the Double Question Mark Operator (??) in JavaScript
The double question mark operator (??) is a powerful tool that can be used to improve the readability, maintainability, and robustness of your JavaScript code. Here are five tips for using the double question mark operator effectively:
Tip 1: Use the double question mark operator to provide default values for variables and properties.
The double question mark operator can be used to assign a default value to a variable or property if the value is null or undefined. This can be useful for preventing errors and ensuring that your code always has a valid value to work with.
const name = user?.name ?? "Guest";
In this example, the double question mark operator is used to assign the default value “Guest” to the name variable if the user object does not have a name property.
Tip 2: Use the double question mark operator to handle null and undefined values in conditional statements.
The double question mark operator can be used to handle null and undefined values in conditional statements. This can help to prevent errors and make your code more robust.
if (user?.name) { // Do something}
In this example, the double question mark operator is used to check if the user object has a name property before executing the code block.
Tip 3: Use the double question mark operator to chain multiple default values.
The double question mark operator can be used to chain multiple default values. This can be useful for providing a fallback value if the first default value is also null or undefined.
const name = user?.name ?? user?.email ?? "Guest";
In this example, the double question mark operator is used to assign the default value “Guest” to the name variable if the user object does not have a name property or an email property.
Tip 4: Use the double question mark operator to simplify error handling.
The double question mark operator can be used to simplify error handling by providing a default value in case of an error.
try { const data = await fetch('https://example.com/api/data');} catch (error) { const data = [];}
In this example, the double question mark operator is used to assign the default value [] to the data variable in case the fetch operation fails.
Tip 5: Use the double question mark operator to enhance the readability and maintainability of your code.
The double question mark operator is a concise and expressive way to handle null and undefined values. This can help to improve the readability and maintainability of your code.
const name = user ? user.name : "Guest";const name = user?.name ?? "Guest";
The second example is more concise and easier to read than the first example.
Summary
The double question mark operator is a powerful tool that can be used to improve the readability, maintainability, and robustness of your JavaScript code. By following these tips, you can use the double question mark operator effectively to handle null and undefined values in your code.
Conclusion
The double question mark operator (??) is a powerful tool that can enhance the quality of your JavaScript code. It provides a concise and expressive way to handle null and undefined values, making your code more readable, maintainable, and robust.
By understanding the purpose, usage, and benefits of the double question mark operator, you can effectively leverage it in your JavaScript applications. This can lead to cleaner, more efficient, and more reliable code that is easier to maintain and debug.
Youtube Video:
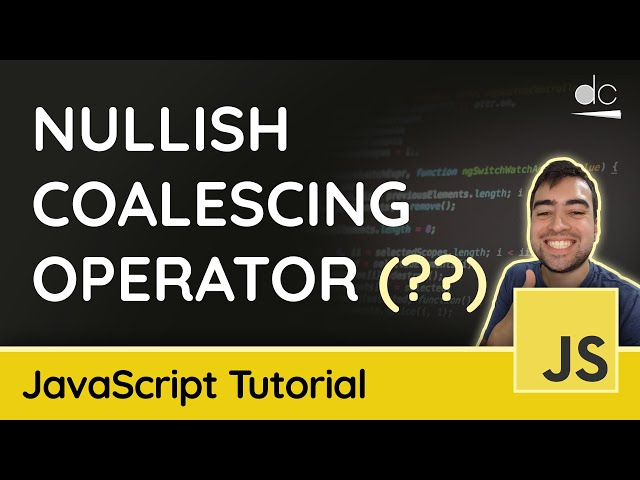